- Topics
- /
- Server-side frameworks
- &Protocols
- /
- Django Channels vs WebSockets: What’s the difference?
Django Channels vs WebSockets: What’s the difference?
When it comes to Django Channels vs WebSockets, figuring out which one to use isn’t always straightforward. Should you use Django Channels if you’re building a realtime application with Django? Is it just another way to implement WebSockets? Or do you need both?
The truth is, Django Channels and WebSockets aren’t direct competitors—they serve different purposes. While WebSockets are a communication protocol for realtime data exchange, Django Channels is a framework built to handle WebSockets (and other asynchronous protocols) within Django applications.
So, which one do you need? Let’s break it down.
What is Django Channels?
Django Channels is a framework that extends Django’s capabilities beyond traditional HTTP, allowing it to handle asynchronous protocols like WebSockets, MQTT, and HTTP2 and enables Django applications to support long-lived connections.
Django itself is synchronous by default, meaning each request is processed one at a time. This is fine for traditional web applications, but it becomes a bottleneck when dealing with realtime use cases that require persistent connections. That’s where Django Channels comes in.
How Django Channels works
Django Channels is built on ASGI (Asynchronous Server Gateway Interface), which is the successor to WSGI. ASGI allows Django to support asynchronous event-driven communication rather than just synchronous HTTP requests. With ASGI, Django Channels can handle multiple simultaneous WebSocket connections, run background tasks outside the request-response cycle, and support event-driven communication across different protocols.
One of the key features of Django Channels is the channel layer—a message-passing system that allows different parts of your application to communicate asynchronously. This is especially useful for managing WebSockets because it enables message broadcasting between different users and workers.
For example, in a chat application, one user sends a message via a WebSocket connection. The message is passed through a channel layer and delivered to all other connected users in the chat room. Django Channels does not store messages itself; it relies on backends like Redis to manage message distribution.
A basic Django Channels architecture might look something like this:
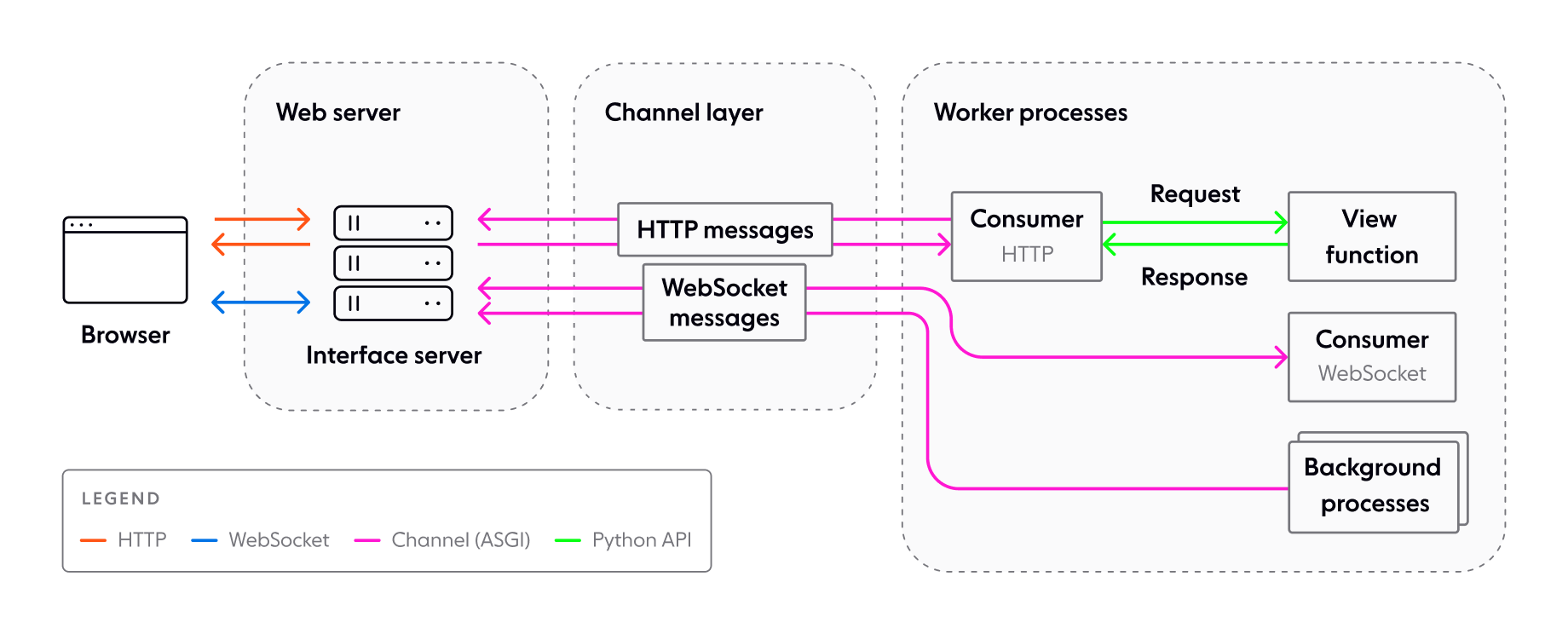
For more information on Django Channels, check out our dedicated guide.
Key features of Django Channels
WebSocket support – Adds WebSocket handling to Django applications.
Multiprotocol support – Works with WebSockets, HTTP2, and MQTT.
Scalability via channel layers – Enables message passing between different Django processes, allowing for distributed WebSocket handling.
Background task execution – Runs asynchronous tasks that don’t block Django’s request-response cycle.
Integration with Django’s authentication and middleware – Allows you to reuse Django’s existing authentication system for WebSocket connections.
Django Channels essentially acts as a bridge that lets Django interact with WebSockets and other asynchronous communication protocols.
What is WebSocket?
WebSocket is a full-duplex communication protocol that enables persistent, bidirectional connections between a client and a server. Unlike HTTP, which follows a request-response model, WebSockets allow data to flow in both directions without needing to establish a new connection each time.
Django Channels makes it possible to use WebSockets within Django applications, but WebSockets themselves are not specific to Django. They are simply a protocol that allows continuous data exchange.
How WebSocket works,
Most applications default to HTTP, but when realtime communication is needed, WebSockets take over. WebSockets actually start as a regular HTTP request before being upgraded to a persistent, bidirectional connection. When a client initiates a WebSocket connection, it sends a request to the server asking if it supports WebSockets. If the server does, the connection is upgraded in a process called the opening handshake. Once established, this WebSocket connection remains open, allowing both the client and server to send and receive messages in realtime with minimal latency—no need for repeated HTTP requests. The connection stays active until either the client or server chooses to close it.
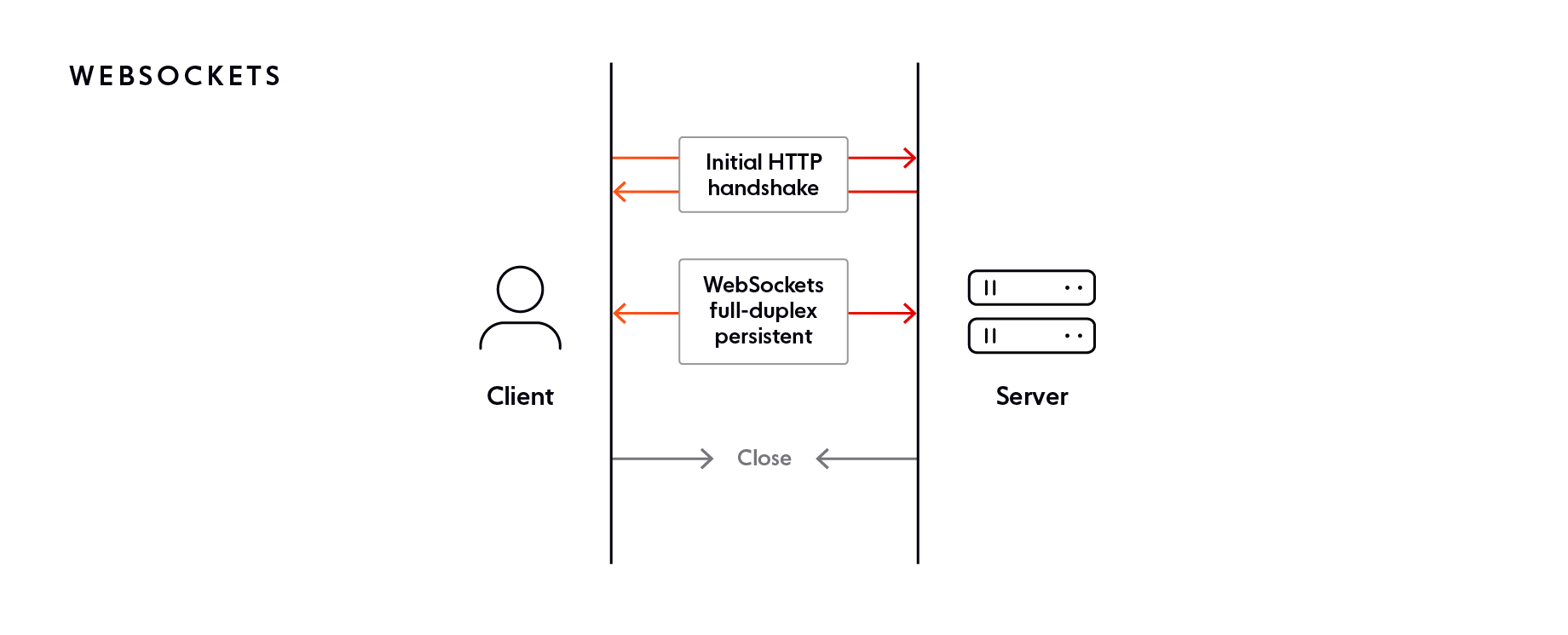
Sequence for a websocket connection/disconnection
Because of its low latency, the WebSocket protocol is typically used in realtime use cases like chat applications, realtime notifications, livestream updates, collaboration tools, and IoT applications. For more information on WebSocket in general, check out our dedicated article.
When to choose Django Channels
Django Channels is the right choice when you're working within the Django ecosystem and need to add WebSocket support or other asynchronous features without adopting an entirely new framework. It’s particularly useful for applications that need multiprotocol support, background task execution, and integration with Django’s authentication and middleware. So, in short, consider Django Channels when:
You’re building a Django application – Django Channels extends Django’s capabilities to support realtime features without requiring a separate WebSocket server.
You need multiprotocol support – Unlike WebSockets alone, Django Channels can handle other asynchronous protocols, including HTTP2 and MQTT.
You require inter-process communication – Django Channels uses a channel layer (such as Redis) to enable message passing between different Django workers, helping to distribute WebSocket connections across multiple processes.
You want to offload tasks from the request-response cycle – It supports background task execution, which can help reduce server load for long-running processes.
You need Django’s authentication and middleware for WebSockets – Django Channels allows you to reuse Django’s built-in authentication system to manage WebSocket connections securely.
However, while Django Channels enables WebSockets within Django, it is not a WebSocket-native solution. Setting up Django Channels requires additional configurations, such as using Redis for the channel layer, and achieving scalability requires extra backend setup with worker pools and proper configurations.
When to choose WebSocket
WebSocket, as a standalone protocol, is the best option when you need low-latency, bidirectional communication at scale, especially if your application is not tied to Django. WebSockets provide persistent, lightweight connections, making them ideal for high-frequency realtime messaging applications.
Consider the vanilla WebSocket protocol in these cases:
You need low-latency, bidirectional communication – WebSockets eliminate the overhead of repeated HTTP requests, providing persistent connections for realtime messaging.
You’re not tied to Django – WebSockets are protocol-agnostic and can be implemented in any framework, making them a flexible choice if you’re not using Django.
You’re building a high-frequency messaging application – Use WebSockets for chat applications, live streaming, stock market feeds, and multiplayer collaboration tools, where message exchange speed is critical.
You have an infrastructure ready for WebSocket scaling – WebSockets require a dedicated WebSocket server as well as load balancing and distributed architectures to handle connections at scale.
However, WebSockets are not natively built into Django, which means using Django Channels or an external service is necessary to integrate them into Django applications. Additionally, scaling WebSocket connections requires careful management of server load, message distribution, and failover strategies.
When to use Django Channels and WebSockets together?
Django Channels is essentially a framework that helps Django applications support WebSockets. If you're using Django and need WebSockets, Django Channels is likely your best option.
However, if you’re dealing with high-scale WebSocket traffic, you may need additional tools to manage load balancing, persistence, and scalability beyond what Django Channels offers.
The key takeaway
Django Channels and WebSockets are not direct competitors, but rather complementary technologies. Django Channels enables Django to handle WebSockets, while WebSockets provide the underlying protocol for realtime messaging. If you're working within Django, Django Channels is often the best approach for integrating WebSockets. However, if you need a more scalable, framework-agnostic WebSocket solution, a managed service can simplify scaling and improve reliability.
Solutions for handling WebSockets at scale
Scaling WebSockets is complex, especially for global applications with large-scale traffic. Django Channels helps, but it still requires additional effort to handle failover, global distribution, and high-throughput messaging. Message ordering and delivery are also not guaranteed. Django Channels is likely to be expensive and hard to manage at scale in-house, just like any other WebSocket-based system. Learn about the challenges of scaling WebSockets.
It’s up to you to decide whether Django Channels is best for your use case, but if you decide the offloading realtime infrastructure is best for your use case at scale, this is where managed realtime services can help you.
At Ably, scalability is baked into what we do. Our platform provides a global pub/sub messaging platform designed for scalable, low-latency realtime communication. With Ably, you get:
Global WebSocket infrastructure for high-availability messaging.
Low-latency messaging (<50ms median latency).
Automatic failover and guaranteed message ordering.
Scalability without the complexity of managing WebSocket servers.
Sign up today to see what Ably can do for you.
Recommended Articles

WebSocket topic page
Get a better understanding of WebSockets are, how they work, and why they're ideal for building high-performance realtime apps.
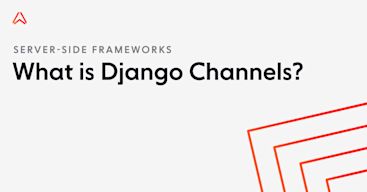
Guide to Django Channels: What it is, pros and cons and use cases
Discover how Django Channels can be used to power realtime experiences like chat and multiplayer collaboration, its pros and cons, and suitable alternatives.
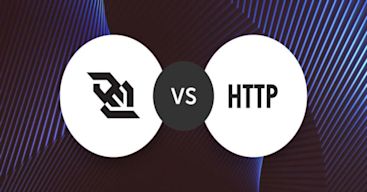
WebSockets vs HTTP: Which to choose for your project in 2024
An overview of the HTTP and WebSocket protocols, including their pros and cons, and the best use cases for each protocol.
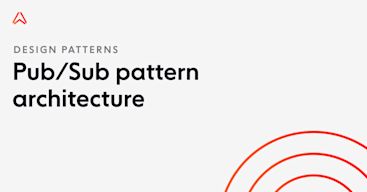
Pub/Sub pattern architecture
Learn about the key components involved in any Pub/Sub system, understand the characteristics of Pub/Sub architecture, and explore its benefits.